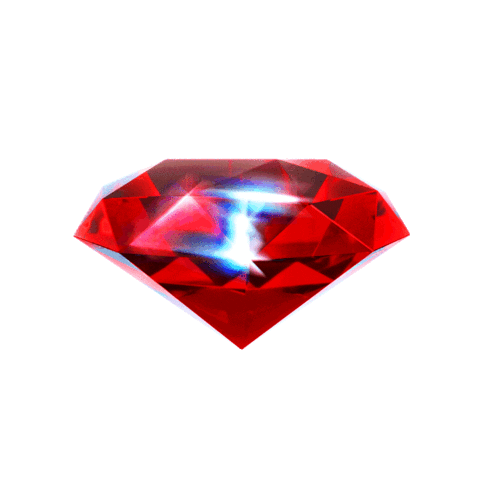

Hello, I am Bunjo, in this topic I will explain how to code a client for the AMQP protocol in Ruby.

AMQP (Advanced Message Queuing Protocol)
AMQP is an abbreviation for Advanced Message Queuing Protocol. This protocol is a network protocol designed to provide reliable messaging between applications.
AMQP is used to exchange, order, route and securely deliver messages.
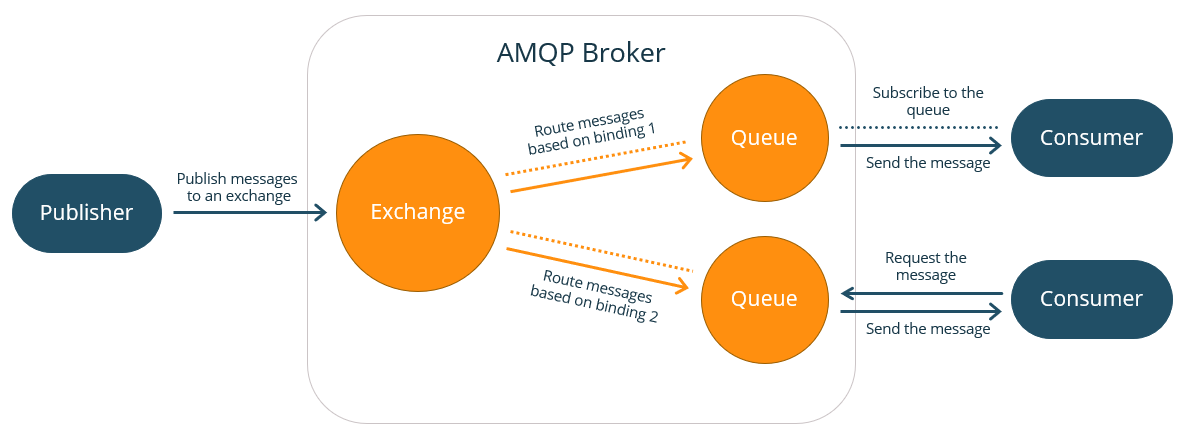
This ensures reliability, flexibility and performance when exchanging data between different applications.
AMQP can be used in many different messaging scenarios, for example in distributed systems, cloud computing environments, and in many industries such as financial services.

History
AMQP was created in 2003 by John O'Hara of JPMorgan Chase in London.[1][7] AMQP was designed as a collaborative open effort. The initial design was done by JPMorgan Chase from mid-2004 to mid-2006, contracting iMatix Corporation to develop a C broker and protocol documentation. In 2005, JPMorgan Chase approached other firms to form a working group that included Cisco Systems, IONA Technologies, iMatix, Red Hat, and the Transaction Workflow Innovation Standards Team (TWIST). That same year, JPMorgan Chase partnered with Red Hat to build Apache Qpid, initially in Java and soon in C++. RabbitMQ was developed independently by Rabbit Technologies in Erlang, followed by Microsoft and StormMQ implementations.
The working group includes 23 members, including Bank of America, Barclays, Cisco Systems, Credit Suisse, Deutsche Börse, Goldman Sachs, HCL Technologies Ltd, Progress Software, IIT Software, INETCO Systems Limited, Informatica (including 29 West), JPMorgan Chase. reached the company. Microsoft Corporation, my-Channels, Novell, Red Hat, Software AG, Solace Systems, StormMQ, Tervela Inc., TWIST Process Innovations ltd, VMware (acquired Rabit Technologies) and WSO2.
In 2008, iMatix's CEO and lead software designer, Pieter Hintjens, wrote an article titled "What's wrong with AMQP (and how to fix it)"[8] and distributed it to his working group to warn of an imminent failure and identify problems . Seen by iMatix and suggested ways to fix the AMQP specification. By then iMatix had already started working on ZeroMQ. In 2010, Hintjens announced that iMatix would leave the AMQP working group and had no plans to support AMQP/1.0 in favor of the significantly simpler and faster ZeroMQ.[9]
In August 2011, the AMQP working group announced its reorganization as an OASIS member chapter.[10]
...
Ref: Advanced Message Queuing Protocol - Wikipedia

Coding a Client with Bunny
Installation:
Bash:
gem install bunny
First, the bunny library is included in the project. This library is used to interact with RabbitMQ.
Ruby:
require 'bunny'
Next, the connection options required to connect to the RabbitMQ server are specified. In this example, the destination IP address and connection port are specified.
Ruby:
options = {
host: 'target ip',
port: 5672
}
The connection is created and initialized.
Ruby:
connection = Bunny.new(options)
connection.start
A channel is created through the connection. The channel is used to communicate with RabbitMQ.
Ruby:
channel = connection.create_channel
A broadcast exchange is created. In this example, an exchange of type fanout named "logs" has been created.
The fanout type sends messages to all connected queues.
The fanout type sends messages to all connected queues.
Ruby:
exchange = channel.fanout('logs')
A message is created. If the user does not enter an argument, it defaults to "Hello World!" message is used.
If arguments are entered, the entered arguments are combined to create a single message.
Ruby:
message = ARGV.empty? ? 'Hello World!' : ARGV.join(' ')
The created message is sent to the broadcast exchange.
Ruby:
exchange.publish(message)
Finally, the information of the sent message is printed to the console.
Ruby:
puts " [x] Sent #{message}"
The connection is closed.
Ruby:
connection.close
Full Code:
Ruby:
# tht bunjo
require 'bunny'
options = {
host: 'target ip',
port: 5672
}
connection = Bunny.new(options)
connection.start
channel = connection.create_channel
exchange = channel.fanout('logs')
message = ARGV.empty? ? 'Hello World!' : ARGV.join(' ')
exchange.publish(message)
puts " [x] Sent #{message}"
connection.close
File Example:
Ruby:
# tht bunjo
require 'bunny'
options = {
host: 'target ip',
port: 5672,
user: 'user',
password: 'pass'
}
connection = Bunny.new(options)
connection.start
channel = connection.create_channel
exchange = channel.direct('my_channel', durable: true)
message = ARGV.empty? ? 'http://target_ip:8080/file.zip' : ARGV.join(' ')
exchange.publish(message)
puts " [x] Sent #{message}"
connection.close
and more than read.